MVCHow Rails Applies the Model-View-Controller Pattern to Process Browser Requests
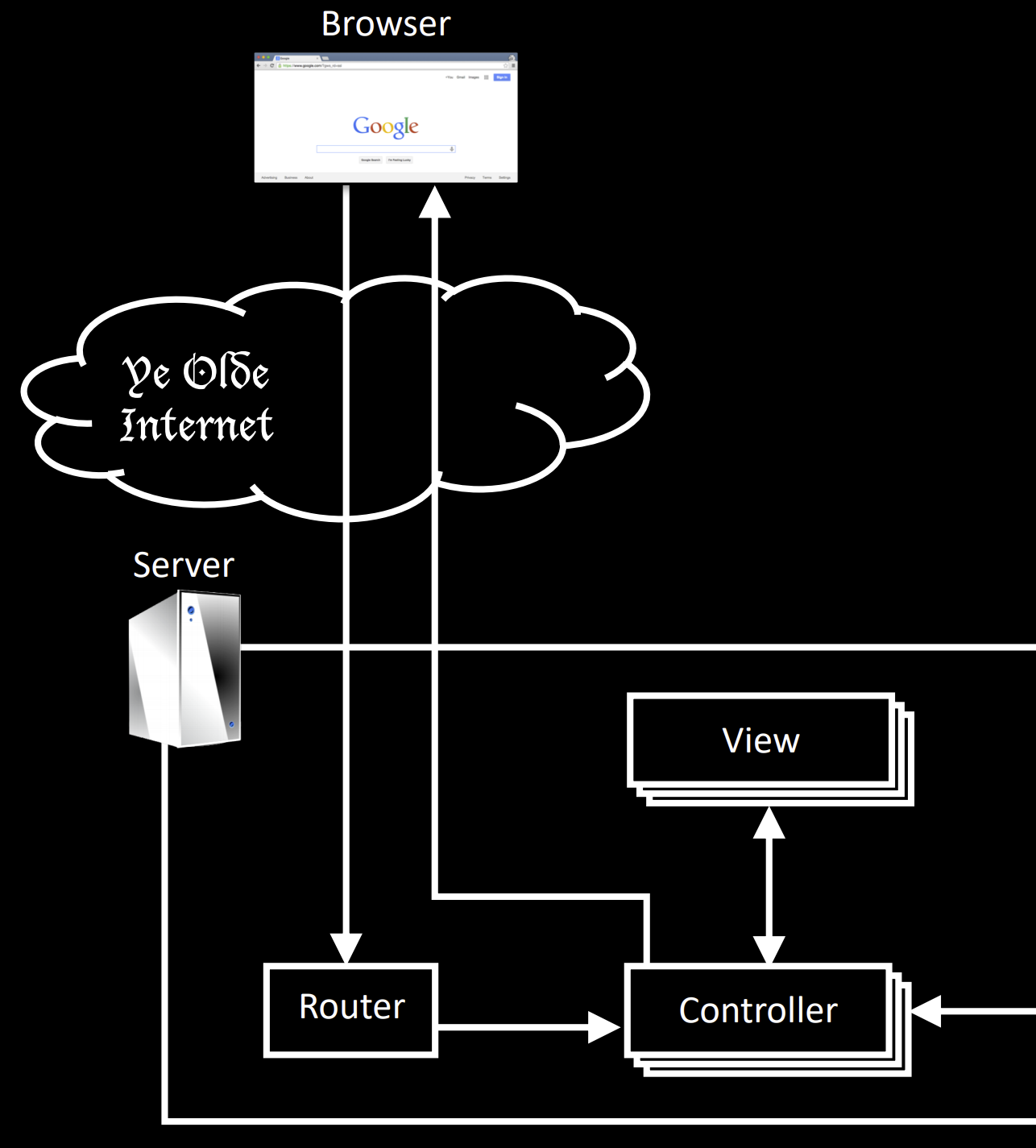
Figure 1. Part of the Rails server architecture.
- User enters URL into browser to generate request
- Server receives request and sends to router
- Router matches request type and URL to controller action
- Controller action renders view
- Server responds to request
Routes
The purpose of a route is to link a URL to a controller action.
The four components of a route are the:
- Request method
- URL pattern
- Controller and controller action names
- Name for the URL pattern
The general form of a route is:
[request_method] "[URL]", to: "[controller]#[action]", as: "[name]" |
The first part, get ‘welcome’ tells the Rails router to accept GET requests to the URL formed by concatenating the site root (for now http://localhost:3000) with a slash (/) followed by the page-specific path in quotes (welcome).
The to
argument tells the router which controller action should be called when it receives such a GET request.
Note that routes use the shorthand “static_pages#welcome” to refer to the method welcome in the StaticPagesController class.
The as
argument automatically generates the helper methods welcome_path and welcome_url, which return the relative path and the full URL for this route, respectively.
You should use these helpers in your code instead of hard-coding paths.
For example, the system root could change (e.g., if the app was deployed to Heroku or was running on a port other than 3000), and using these helpers will save you from having to search for and update all the hard-coded URLs spread throughout your code.
Controller
A controller is a ruby class made up of methods, also called actions because they perform actions in response to a server request.
Actions can have three main responsibilities:
- Act on model objects
- Render views
- Redirect to other controller actions
Views
Rails views use the .html.erb
extension instead of .html
, so they can run embedded ruby code.